How Do I Properly Connect Apps and APIs? A Simple, Powerful Guide for Beginners in 2025
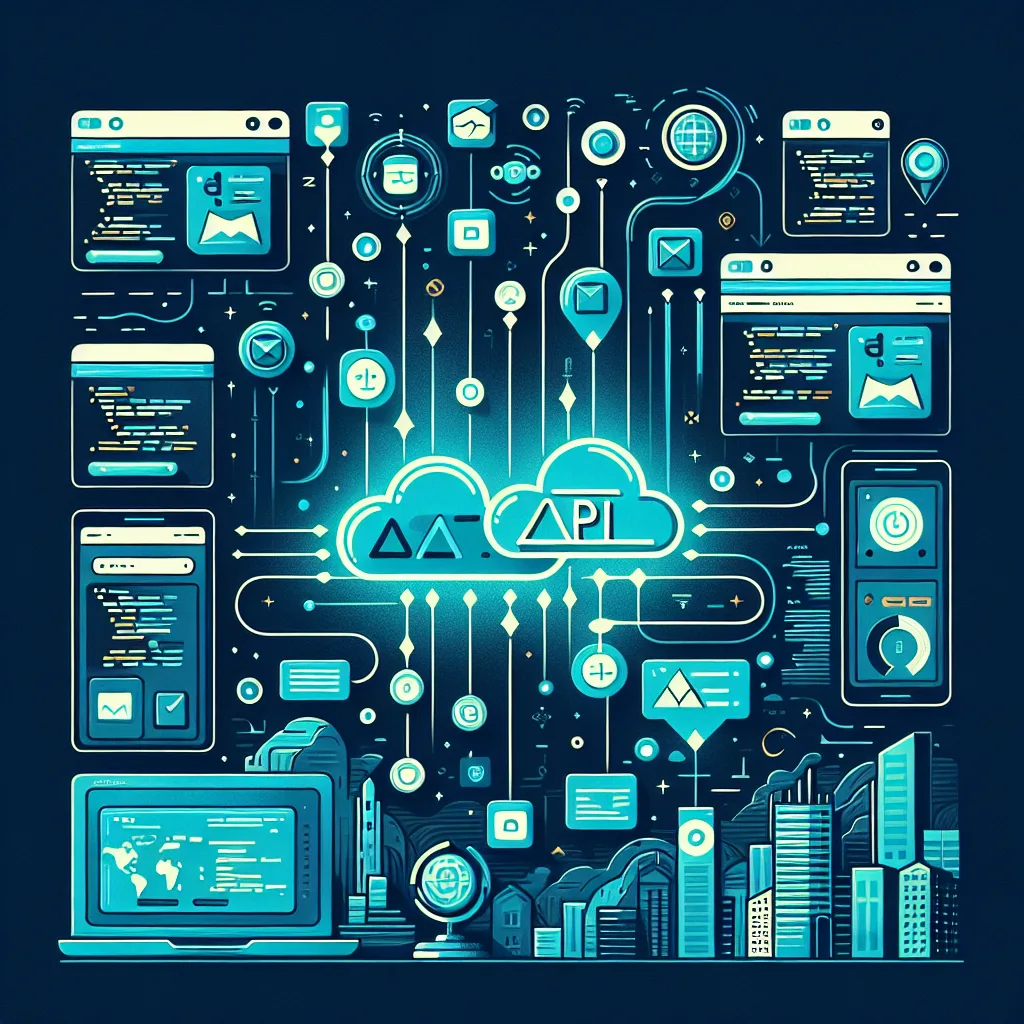
Table of Contents
Learn how to connect apps and APIs the right way—even if you’re not a developer. A simple, beginner-friendly guide with real examples and tools to get you started.
What Is an API, Really?
Let’s break it down in human terms:
An API (Application Programming Interface) is like a waiter in a restaurant.
You (the app) ask the waiter (API) for something — maybe the weather in New York. The waiter goes to the kitchen (external service), grabs the info, and brings it back neatly on a plate (in code form).
Pretty neat, right?
Why Apps and APIs Need to Connect
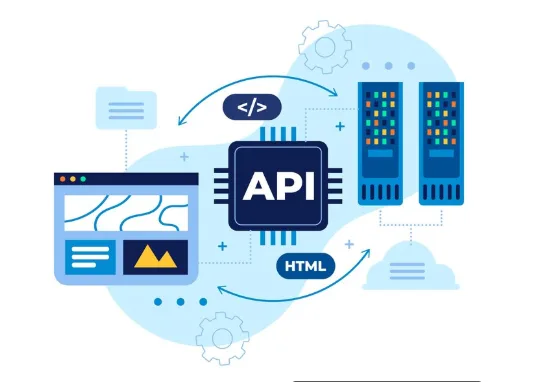
Apps can’t do everything on their own. So they call on APIs to:
- Get real-time weather data (e.g., OpenWeather)
- Verify user login with Google
- Process payments via Stripe
- Post social media updates on X (Twitter)
- Send email notifications with Mailgun or SendGrid
- Fetch product inventory from Shopify or Amazon
In short: APIs are bridges between your app and the powerful tools on the web.
Tools You Need to Connect an API
Here’s a quick checklist before you begin:
Tool | Why You Need It |
---|---|
🔧 Code editor (VS Code) | To write and test your code |
📘 API documentation | Your instruction manual |
🔐 API key or token | Unique ID to authenticate your app |
🌍 Internet access | APIs live online |
🧪 API testing tools (Postman, Insomnia) | To test before coding |
📦 Package managers (npm, pip) | To install useful API libraries |
How to Properly Connect an App to an API (Step-by-Step)
Let’s walk through a real-life example of connecting a weather API using JavaScript. These steps apply to nearly any API integration.
Step 1: Choose the Right API
Pick an API that fits your need. Let’s say you want weather data — you might go for:
Check if the API is:
- Free to use (or has a free tier),
- Well-documented,
- Actively maintained.
Step 2: Get Your API Key
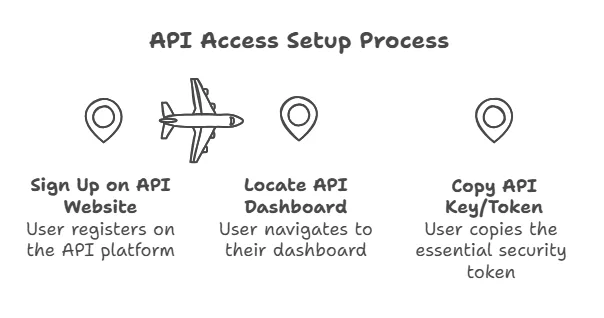
Tip: Never share this key in public code (like GitHub). Use .env
files or secret managers to store them safely.
Step 3: Read the API Documentation
Documentation is your map. Look for:
- Base URL (e.g.,
https://api.openweathermap.org
) - HTTP methods used (GET, POST, PUT, DELETE)
- Required headers, parameters, and authentication
- Example responses (usually in JSON format)
Step 4: Test the API in Postman or Insomnia
Before writing code, open Postman and:
- Paste the API URL.
- Add your parameters and headers.
- Click Send.
If it returns a valid response, you’re ready to code.
Step 5: Write the API Call in Code
Here’s a basic JavaScript example using fetch()
to connect to OpenWeather:
javascriptCopyEditconst city = "New York";
const apiKey = process.env.OPENWEATHER_API_KEY;
const url = `https://api.openweathermap.org/data/2.5/weather?q=${city}&appid=${apiKey}`;
fetch(url)
.then(response => {
if (!response.ok) throw new Error("API error");
return response.json();
})
.then(data => console.log("Weather data:", data))
.catch(error => console.error("Error:", error));
You can do the same in:
- Python (with
requests
) - PHP (with
curl
) - Node.js (with
axios
)
Step 6: Handle Errors and Failures
Always assume something can go wrong:
- API limit reached?
- Wrong parameters?
- Network down?
Example error handling:
javascriptCopyEditif (!response.ok) {
throw new Error(`Error: ${response.status}`);
}
Show user-friendly messages, and log the details silently.
Step 7: Secure Your API Keys
Use:
.env
files for local development,- GitHub secrets or AWS Secrets Manager in production,
.gitignore
to prevent leaking secrets.
Bonus: Test with Postman First
Before writing code, try your API call in Postman. It helps you understand:
- Request headers
- Parameters
- Response format
Pro Tips for Better App + API Connections
- Use axios instead of
fetch
for cleaner syntax (in JS projects) - Use retry logic for unstable APIs
- Cache frequent responses (e.g., with localStorage or Redis)
- Respect rate limits — too many requests can block your key
- Use OAuth if the API needs login (e.g., Google, GitHub)
Common Mistakes to Avoid
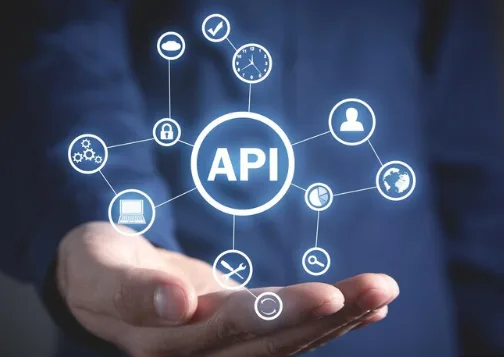
Even experienced developers run into issues when working with APIs. To help you avoid the most common pitfalls, here are some mistakes you should watch out for — and how to fix them:
- Exposing API Keys in Public Repositories
One of the biggest security mistakes is accidentally uploading your API keys to platforms like GitHub. These keys are like passwords, and if someone else gets access, they can misuse your account. Always store them in environment variables (.env
files) and use a.gitignore
file to prevent them from being exposed.
- Ignoring API Documentation
Skipping or skimming the documentation is a fast way to run into errors. Every API has its own structure, required parameters, methods, and rate limits. Take the time to read the documentation thoroughly — it’s your best friend when working with APIs.
- Not Handling Errors Properly
APIs don’t always work as expected. Servers go down, data formats change, or requests can time out. Always include proper error handling in your code usingtry/catch
or.catch()
blocks. Show user-friendly error messages and log the technical details for debugging.
- Hardcoding API URLs or Keys in Code
Avoid writing sensitive information like URLs or API keys directly into your code. It makes your app less flexible and more vulnerable. Instead, keep them in configuration or environment files, and reference them securely.
- Ignoring Rate Limits and Quotas
Most APIs have limits on how many requests you can send in a given time (like 60 per minute or 10,000 per month). If you exceed these, your app may stop working temporarily or get blocked. Always check the rate limits in the documentation and implement throttling or retries if needed.
- Using the Wrong HTTP Methods
Each API endpoint requires a specific method — like GET, POST, PUT, or DELETE. Using the wrong one can result in errors or data loss. Always verify the correct method for each endpoint in the API docs.
- Skipping Response Validation
Just because you got a response from the API doesn’t mean the data is valid or complete. Always check if the response contains the expected structure or fields before using it in your app. This avoids bugs and broken displays.
- Not Testing API Calls Before Coding
Jumping straight into coding without testing the API can waste hours. Use tools like Postman or Insomnia to test requests first. This ensures your URL, parameters, and authentication are correct before you write a single line of code.
- Not Using Secure Connections (HTTPS)
Always use HTTPS when making API requests. It encrypts the data and keeps user information safe. Many APIs won’t even accept non-HTTPS connections, so it’s essential for both security and functionality.
- Forgetting Pagination for Large Datasets
Some APIs return data in chunks or “pages” to avoid overwhelming your app. If you don’t implement pagination, you might miss data or crash your app by trying to load everything at once. Always check if the API response includes pagination info and handle it accordingly.
Mistake | Solution |
---|---|
Exposing API key in code | Use environment variables |
Not reading docs | Always review endpoint requirements |
Not handling errors | Add .catch() blocks |
Hardcoding URLs | Use config files |
Ignoring rate limits | Implement retry and throttle logic |
Final Thoughts:
In today’s fast-moving digital world, knowing how to properly connect apps and APIs is like learning a superpower.
It transforms a basic app into a dynamic, real-time platform connected to the world. Whether you’re a solo developer, a startup founder, or just curious — APIs are the key to unlocking possibilities.
So go ahead — start connecting, start creating, and watch your app come to life!
FAQs:
1. What is an API in simple terms?
An API (Application Programming Interface) allows two different applications or systems to talk to each other. For example, when you use a weather app, it pulls real-time weather data from a remote server using an API. Think of it like a digital waiter taking your order and bringing back the dish.
2. Do I need to know coding to use APIs?
Some basic coding knowledge is helpful — especially in languages like JavaScript, Python, or PHP. However, if you’re using no-code platforms like Zapier or Make (Integromat), you can connect apps using pre-built API modules without writing any code.
3. How do I get an API key?
Most APIs require you to create a free account on the service’s website. Once signed in, you can usually find your API key under a section like “Developer,” “API Access,” or “Integrations.” Keep your API key private and secure — it acts like a password.
4. What is the difference between REST API and SOAP API?
REST is a simpler, more modern style of API that works with standard HTTP methods like GET and POST. SOAP is older and more rigid, using XML for requests. Today, REST APIs are more popular and easier for beginners to work with.
5. Can I connect any two apps using APIs?
In most cases, yes — if both apps offer APIs. The challenge lies in understanding how each API works and whether their data formats are compatible. That’s where middleware tools like Zapier, Pipedream, or custom scripts come in handy.
6. What happens if I exceed the API rate limit?
If you go over the rate limit (i.e., too many requests in a short time), your app may receive a “429 Too Many Requests” error or get temporarily blocked. Always check the API documentation and implement error handling and retry logic to stay within limits.
IF YOU REACH AT END, GO GIVE A LOOK AT blogs ON OUR MAIN website.